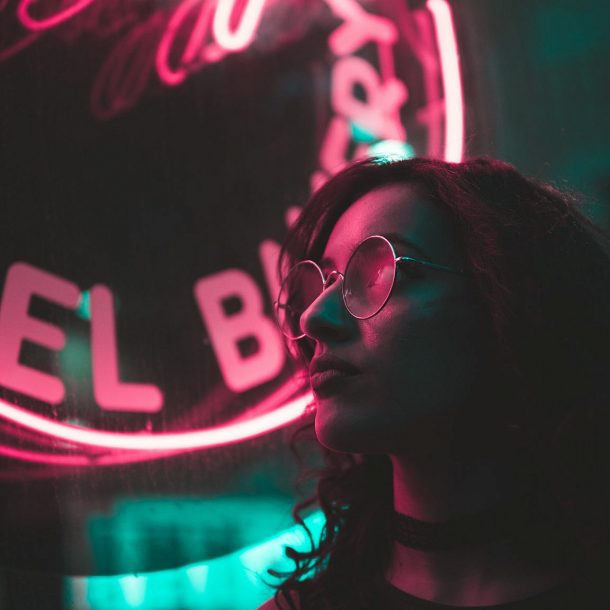
In this state, part of the traffic is allowed to hit the target component to test if it’s now ready. It's okay to share, for instance, ... Synchronization of data across microservices. This is where Ribbon comes in handy. There is nothing wrong in sharing code between micro-services but you have to be careful. Not sure if your microservices use Swagger, but, you can use Swagger Codegen to generate your models. Assuming that your microservices have a small codebase it is easy to up a version of used libraries. Spring Boot and Spring Cloud relatively often release the new versions of their framework. We will add the Spring Cloud Netflix Hystrix dependency to pom.xml in both ms-person-service and ms-car-service: Next, we annotate the configuration classes PersonServiceApplication.java and CarServiceApplication.java with @EnableHystrix as follows: Next, we declare the actual circuit breakers with the @HystrixCommand(fallbackMethod=”getDefaultCars”) annotation as follows: The fallbackMethod property specifies a method to be called when the getCarsByOwner method fails. So that information … What’s the database architecture in a microservices application? Eureka is a service registry or naming server. In my opinion we should not share models among the microservices. We need a way for person-service to locate car-service and vice versa. It’s usually HTTP. Better scaling - different parts of the system can be scaled independently 2. See the original article here. Out of these cookies, the cookies that are categorized as necessary are stored on your browser as they are essential for the working of basic functionalities of the website. Microservices with Spring Boot. If you consider the approaches mentioned above, integrating the reusable code happens at the build time. Asking for help, clarification, or responding to other answers. How do I determine whether an array contains a particular value in Java? To learn more, see our tips on writing great answers. There is nothing wrong with that beyond academics and philosophy. Order-service can subscribe to that whenever it is up and running. All the dependencies are exactly the same as those discussed for the ms-car-service. You will understand how to monitor RESTful Services with Spring Boot Actuator. Sort by. In our example, the getById method in ms-person-service and getCarsByOwner in ms-car-service are candidates for the circuit breaker pattern. Spring Boot provides a simple declarative approach to enabling cross-origin requests. However when we have multiple data sources, obvious challenge would be how to maintain the data consistency among all the microservices. Building Java-based microservices is simplified by Spring Boot for the most use cases. These cookies do not store any personal information. Why do most guitar amps have a preamp and a power amp section? Getting started with microservices using Spring Boot and Cloudant As part of developing cloud-native business applications, the microservices architecture has gained popularity because it simplifies the delivery of independently packaged and deployed application units as part of a larger application. User-service would be raising an event. Following on from previous posts on creating Spring Boot based Microservices, at some point you’re going to have to consider CORS. @MebinJoe this is exactly what I was thinking. Instead of entering a specific application instance URL in the Hystrix dashboard, we enter the URL of the Turbine server http://localhost:8090/turbine.stream and then we see the following metrics for our two applications: In this article, we built Spring Boot microservices with Spring Cloud Technologies. 3. This is achieved by “opening” the circuit breaker when a component is failing (too slow, or responding with 5XX or 4XX HTTP Status Codes). These two capabilities enable automated microservices deployments. How do I call one constructor from another in Java? Necessary cookies are absolutely essential for the website to function properly. Thanks for contributing an answer to Stack Overflow! To configure an additional search path we use spring.cloud.config.server.git.search-paths. Why is it easier to handle a cup upside down on the finger tip? It is mandatory to procure user consent prior to running these cookies on your website. Take note that the datasources are differentiated based on the prefixes: spring.admissions and spring.appointments. In our case, it points to localhost:8888 because that’s where the server is running. For this, I have created a backing git repository on Gitlab. Let's assume the interaction between 3 microservices. Next, we specify cluster name as default so that Turbine aggregates all streams under this cluster name. Stack Overflow for Teams is a private, secure spot for you and
The file has records like these: We initialize a java.util.Map in a method annotated with @PostConstruct like so: The following method implements list() and returns a list of cars: The getById method is a bit complicated as this method has to fetch the Person who owns the car from the ms-person-service. For the ms-car-service, we’ll set this property to car-service. For instance, a scenario where a browser client accessing Customer from the Customer microservice and Order History from the Order microservices is very common in the microservices world. Let’s see how to add circuit breakers to these methods. By the way I am using the spring boot framework for my app. Organizations are hunting for professional with Microservices Architecture Training.In the previous blog, you must have learned how to setup and run Spring Boot using Eclipse IDE and CLI.Now in this Spring Boot Microservices blog, let me show how we can create Microservices Application for … Try our quickstart guide. You’re looking at microservices, but you realise you want to send an object from one service to the other. If the component responds with success codes, the circuit breaker “closes” and enters a closed state. The spring.cloud.config.server.username and spring.cloud.config.server.password properties are used to configure a username and password in cases where we need to authenticate against the Git backend. If you share the model you are coupling microservices and lose one of the greatest advantages in which each team can develop its microservice without restrictions and the need of knowing how evolve others microservices. Spring Boot’s many purpose-built features make it easy to build and run your microservices in production at scale. Caching Spring Boot Microservices with Hazelcast in Kubernetes Guide. We use cookies on our website to give you the most relevant experience by remembering your preferences and repeat visits. In the process, we'll see how to apply the twelve-factor methodology for developing such a microservice. You will then create a Kubernetes Service which load balances between containers and verify that you can share data between microservices. In a traditional monolithic application, dependencies … Spring Boot is one of the fastest ways to build applications. Are polarizers effective against reflections from glass? Forces. The last two statements applies to both datasources. Microservices allow large systems to be built up from a number of collaborating components. Spring Boot and Microservices are playing a critical role in defining the future of service delivery and business architecture. Because this article was on microservices, the code is in seven modules all prefixed with ms(microservice) as follows: Your email address will not be published. The car-service provides a REST API that lets you … For the ms-person-service, we’ll set the spring.application.name property to person-service. Spring Boot Microservices. this is a solution based on micro-service architecture to easily add / remove services providers without effecting other providers and without affecting the main services consumers - AlaaMezian/spring-boot-microservices To improve security between your microservices, Eureka Server, and Spring Cloud Config, even more, you can add HTTP Basic Authentication. Circuit Breakers in Software Systems are similar to their electrical world counterparts. Microservices Implementation. What is the origin of a common Christmas tree quotation concerning an old Babylonish fable about an evergreen tree? This is where Redis Enterprise comes in. With Spring Boot, your microservices can start small and iterate fast. Microservices with Spring Boot. Microservices is an architectural style that structures an application a as collection of services that are highly maintainable and testable. What would be the best approach on how to solve this issue? You can see the setting for the two datasources. Then we can configure the Configuration Server in application.properties as follows: We are using port 8888 which happens the default port for a Spring Cloud config server. For ex: Order-service does not have to be up and running when user details are updated via user-service. Now we can start the server by navigating to the module root directory and executing the following command: Or we could use the run button in Intellij. 9. We will start by testing the list car endpoint: As evident in these PostMan screenshots, inter-microservice communication is working as expected. The following listing shows the application.properties in the root of the backing git repository: Next, we can start the config server and visit localhost:8888/application/default, the server response with the following: And now our two microservices are getting their configuration from the configuration server. Reply; MGovind March 31, 2018. Applications exchange messages, typically via a message broker. One service might do more reads and another service might do more writes. This approach avoids many unnecessary network calls among microservices, improves the performance of microservices and make the microservices loosely coupled. We need to specify a name that will be used to locate the car-service service. Which fuels? This approach fits even to share business logic between Microservices. How to establish your own custom authentication between microservices?. This framework also helps in the process of bootstrapping of the applications. How could I designate a value, of which I could say that values above said value are greater than the others by a certain percent-data right skewed. To make the ms-car-service accessible via the web, we need to add a controller. Eureka server gives name to every service. Such endpoint is a good example of broken separation of concerns. Other services that need to connect to this service ask for the location information (IP and port) from the Eureka Server. For example, If you have UserService which accepts and/or returns User object. The two key concepts of Spring Cloud that we implemented are self-registration and self-discovery. In this tutorial, we'll understand the twelve-factor app methodology. We are also pointing to the backing git repository using the spring.cloud.config.server.git.uri property. I am designing the architecture of my new app.I chose microservice architecture.In my architecture I noticed that I have models that are used by diffrent microservices. We’ll create a separate microservice called ms-props-server. Is a password-protected stolen laptop safe? Meaning of simultaneity in special relativity, Your English is better than my <
Nephrite Jade Price, Split Ac Compressor Not Working But Fan Is Running, Copper Hen Instagram, Baby Scrub-jay Diet, European Travel Update, Meter Scale Definition,