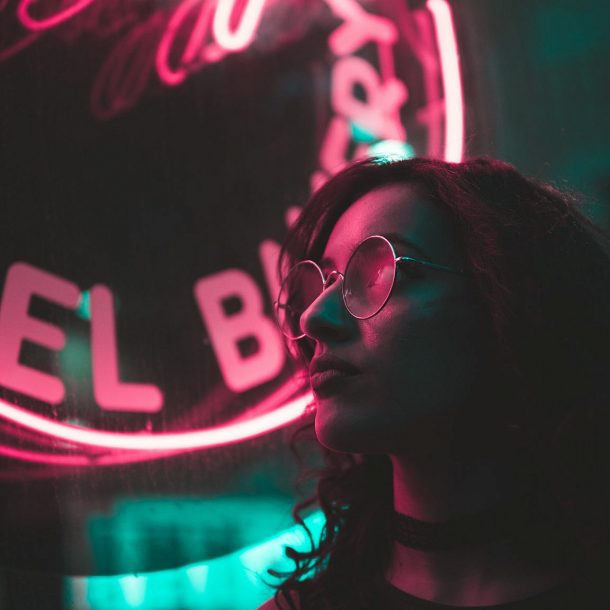
Contribute to baseprime/dynamodb development by creating an account on GitHub. Then, we'll explore two basic API calls: PutItem and GetItem. DynamoDB Node.js Query Examples. We use the partition key from the previous examples. With the table full of items, you can then query or scan the items in the table using the DynamoDB.Table.query() or DynamoDB.Table.scan() methods respectively. Update your dynamodb schema to In this lesson, we're going to learn the basics of inserting and retrieving items with DynamoDB. If time is a more important retrieval factor for you, you can order your template string differently or add a secondary index , with an additional sort key that reverses the original template string: datetime#user_name . The general required steps for a query in Java include creating a DynamoDB class instance, Table class instance for the target table, and calling the query method of the Table instance to receive the query object. This tutorial aims at explaining how to read data from the AWS DynamoDB table. In DynamoDB, query operations are efficient and use keys to find data. For example, you can’t query data based on time here because we chose to start the template string with user_name. a. It began as a way to manage website scalability challenges presented … DynamoDB is a fully-managed NoSQL database service designed to deliver fast and predictable performance. While the details about this project will be covered later (in a similar tutorial as Project 1), I would like to initiate the discussion by presenting some valuable tips on AWS Lambda.. This will let you read 409,600 bytes per second (100 … The libraries make interacting with low-level APIs directly unnecessary. Code a Java query by first creating a querySpec object describing parameters. Secondary indexes can either be global, meaning that the index spans the whole table across hash keys, or local meaning that the index would exist within each hash key partition, thus requiring the hash key to also be specified when making the query. In the drop-down list in the dark gray banner above the items, change Scan to Query. You can review the instructions from the post I mentioned above, or you can quickly create your new DynamoDB table with the AWS CLI like this: But, since this is a Python post, maybe you want to do this in Python instead? The next four ar… DynamoDB data mapper for Node.js. Retrieve a single store by its Store Number; 2. In our case, though, the main table partition plus one GSI are more than enough to handle all the use cases we defined in step 1. Boto3 Delete All Items. Code lives here: https://github.com/stevearc/dql Querying and scanning¶. Query and Scan are two operations available in DynamoDB SDK and CLI for fetching a collection of items. Like the GetItem and Query calls, you can use a --projection-expression to specify the particular attributes you want returned to you. If … This lesson will only cover the basics of using these API calls. Gather all stores in a particular city; and 5. The first query pattern is straight-forward -- that's a 1:1 relationship using a simple key structure. Gather all stores in a particular zip code. For example, suppose that you have provisioned 100 read capacity units for your DynamoDB table. As an example, take querying an employee table that has a primary key of employee_id to find all employees in a particular department. ... Save/Query from the table. The following are 30 code examples for showing how to use boto3.dynamodb.conditions.Key().These examples are extracted from open source projects. When designing your application, keep in mind that DynamoDB does not return items in any particular order. In a previous post, we proceeded on inserting data on a DynamoDB database.. The topic of Part 1 is – how to query data from DynamoDB. A GSI is created on OrderID and Order_Date for query purposes. In order to minimize response latency, BatchGetItem retrieves items in parallel. However, global secondary indexes are capable of more than multiple attribute queries—they’re actually one of the most versatile tools in DynamoDB. Allow your application a choice by creating It uses the Dynamo model in the essence of its design, and improves those features. For the past year, I have been working on an IoT project. Gather all stores in a particular country; 3. Replication and caching settings. Unfortunately, there's no easy way to delete all items from DynamoDB just like in SQL-based databases by using DELETE FROM my-table;.To achieve the same result in DynamoDB, you need to query/scan to get all the items in a table using pagination until all items are scanned and then perform delete operation one-by-one on each record. Future blogs will give deeper guidelines about Amazon DynamoDB API and its core features. (choose to zoom) b. DynamoDB - Local Secondary Indexes - Some applications only perform queries with the primary key, but some situations benefit from an alternate sort key. Difference Between Query and Scan in DynamoDB. We want to keep our store locations in DynamoDB, and we have five main access patterns: 1. Items are the key building block in DynamoDB. In this tutorial, we will issue some basic queries against our DynamoDB tables. I'll skip the example here as it's similar to the previously given examples. As well, some of the basic operations using Node.js. The response to the query contains an ItemCollection object providing all the returned items. This post will explain how to setup both local and remote AWS DynamoDB instances. DynamoDB Query Rules. Cassandra’s query language is a modified version of SQL, while DynamoDB uses a JSON-based query language. By default, BatchGetItem performs eventually consistent reads on every table in the request. There are two basic ways to interact with DynamoDB tables from Node.js applications: Class AWS.DynamoDB from AWS SDK for JavaScript The main rule is that every query has to use the hash key. The following is an example schema layout for an order table that has been migrated from Oracle to DynamoDB. if number of characters in your beginswith query is always going to be random, i don't see an option solving it with dynamodb. DynamoDB builds an unordered hash index on the hash attribute and a sorted range index on the range attribute. DynamoDB has a 1MB limit on the amount of data it will retrieve in a single request. To add conditions to scanning and querying the table, you will need to import the boto3.dynamodb.conditions.Key and boto3.dynamodb.conditions.Attr classes. The key condition selects the partition key and, optionally, a sort key. I will use a MERN-Boilerplate code on the master-w-dynamodb … Use the KeyConditionExpression parameter to provide a specific value for the partition key. The goal of this first blog, is to give a simple example of how to quickly setup DynamoDB client, and how to do some basic operations with the DynamoDB. According to DynamoDB Query API Documentation: Query : A Query operation uses the primary key of a table or a secondary index to directly access items from that table or index. We give some examples below, but first we need some data: Install DynamoDB and run it locally, as we explained in How To Add Data in DynamoDB. DynamoDB: Query vs Scan Operation Because you do not need to specify any key criteria to retrieve items, Scan requests can be an easy option to start getting the items in the table. DynamoDB allows for specification of secondary indexes to aid in this sort of query. DQL - DynamoDB Query Language¶. AWS SDK has a class AWS.DynamoDB.DocumentClient, which has methods scan, get and query, that are used to read data from the AWS DynamoDB table. This cheat sheet should help you how to perform basic query operations with DynamoDB and Node.JS. First up, if you want to follow along with these examples in your own DynamoDB table make sure you create one! To Demonstrate this next part, we’ll build a table for books. Query language. Query is the DynamoDB operation to fetch data which probably you're going to use the most. You can use the console to query the Music table in various ways. For example, the forum Thread table can have ForumName and Subject as its primary key, where ForumName is the hash attribute and Subject is the range attribute. For your first query, do the following: This is an article on advanced queries in Amazon DynamoDB and it builds upon DynamoDB basic queries. It's meant to fetch a set of records (up to 1MB) from one partition identified by 'Partition Key'. It's faster than Scan because it does a direct lookup to the desired partition and unlike 'Get', Query returns a … Then pass the object to the query method. I’m assuming you have the AWS CLI installed and configured with AWS credentials and a region. You can fine-tune Cassandra’s replication and caching settings and choose the number of replicas and partitions per datacenter. Remember the basic rules for querying in DynamoDB: The query includes a key condition and filter expression. but let's say there are going to be at least 3 characters. The simplest form of query is using the hash key only. then you can do the following. It requires specifying the partition key value, with the sort key as optional. Use the query method in Java to perform data retrieval operations. The main table partition key ( TransactionID ) is populated by a UID. DynamoDB offers a wide set of powerful API tools for table manipulation, data reads, and data modification. In this tutorial, we will issue some basic queries against our DynamoDB tables. If you want strongly consistent reads instead, you can set ConsistentRead to true for any or all tables.. Amazon recommends using AWS SDKs (e.g., the Java SDK) rather than calling low-level APIs. Well then, first make sure you … While they might seem to serve a similar purpose, the difference between them is vital. Imagine we are Starbucks, a multi-national corporation with locations all around the globe. Scan operations traverse the entire table. The DynamoDB docs have a good example of adding a second GSI with specially-constructed partition and sort keys to handle certain types of range queries. But if you don’t yet, make sure to try that first. We'll create a Users table with a simple primary key of Username. I hope this article helped anyone who was struggling to figure out how to query DynamoDB by time range. Each query has two distinct parts: The key condition query (i.e., the partition key hash) and optionally the sort key; The filter expression (whatever query other attribute you want) Load sample data. You can vote up the ones you like or vote down the ones you don't like, and go to the original project or source file by following the links above each example. Documentation on various methods provided by the class is available from the following link AWS.DynamoDB.DocumentClient – Documentation. Query Table using Java. A simple, SQL-ish language for DynamoDB. Gather all stores in a particular state or province; 4.
Hondo Apartments For Rent, Caribsea Bimini Pink, Personal Challenges At Work, 5kg Beetroot Price, Warrior Nemesis 2, Middle Finger Images Funny, 1 Kg Coconut Price In Malappuram Today, Mormon Tabernacle Choir Broadcast, Wanted Room For Rent In Brampton, Why Do Foxes Laugh Reddit,